一. 继承图
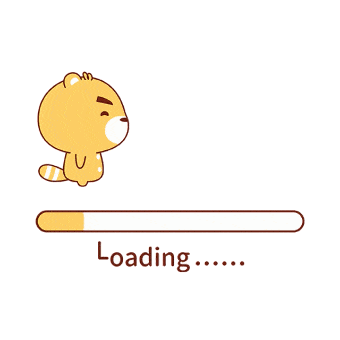
二. Xml方式
1. 所有的布局共有的属性
① View在左上右下四个方向和其他View之间的距离,值是 dp
1 2 3 4
| android:layout_marginStart (android:layout_marginLeft) android:layout_marginTop android:layout_marginEnd (android:layout_marginRight) android:layout_marginBottom
|
② View内部元素到View左上右下边框之间的距离,值是 dp
1 2 3 4
| android:paddingStart (android:paddingLeft) android:paddingTop android:paddingEnd (android:paddingRight) android:paddingBottom
|
③ 容器 内部的对齐方式 以及 容器相对于父容器的对齐方式
1 2
| android:gravity android:layout_gravity
|
2. RelativeLayout 独有的属性
① 相对父容器位置,值是 true 或者 false
1 2 3 4
| android:layout_alignParentStart (android:layout_alignParentLeft) android:layout_alignParentTop android:layout_alignParentEnd (android:layout_alignParentRight) android:layout_alignParentBottom
|
② 相对其它View位置,值是 其它View 的 id值
1 2 3 4
| android:layout_alignStart (android:layout_alignLeft) android:layout_alignTop android:layout_alignEnd (android:layout_alignRight) android:layout_alignBottom
|
③ 在其它View的上(下)边,值是 其它View 的 id值
1 2
| android:layout_above android:layout_below
|
④ 相对于 父容器,水平方向,垂直方向是否居中,值是 true 或者 false
1 2 3
| android:layout_centerInParent android:layout_centerHorizontal android:layout_centerVertical
|
⑤ 在指定视图的上下方
1 2
| android:layout_below="@+id/XXX" android:layout_above="@+id/XXX"
|
3.简单的demo
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47
| <?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity">
<RelativeLayout android:layout_width="300dp" android:layout_height="300dp" android:background="@color/colorAccent" android:layout_centerInParent="true">
<Button android:id="@+id/btn1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="按钮1" android:layout_alignParentBottom="true" android:layout_alignParentEnd="true" android:layout_marginBottom="10dp" android:layout_marginEnd="10dp" />
<Button android:id="@+id/btn2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="按钮2" android:layout_alignBottom="@id/btn1" android:layout_alignParentStart="true" android:layout_marginStart="10dp" android:paddingStart="30dp" />
<Button android:id="@+id/btn3" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="按钮3" android:layout_above="@id/btn1" />
</RelativeLayout>
</RelativeLayout>
|
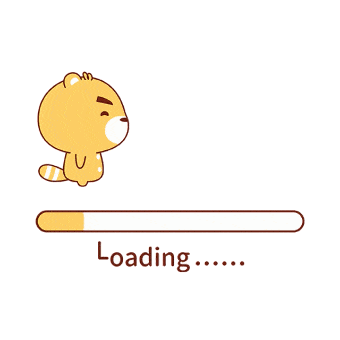
三. Java代码
1.操作步骤
1.找到 View 想要添加的容器
2.创建 View ,并设置相关属性
3.创建对应容器类型的 LayoutParams,根据自己的要求设置约束
2.操作例子
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| RelativeLayout rootLayout = (RelativeLayout) findViewById(R.id.root_layout);
XLImageView dotImgView = new XLImageView(getApplicationContext());
RelativeLayout.LayoutParams relativeParams = new RelativeLayout.LayoutParams( ViewGroup.LayoutParams.WRAP_CONTENT, ViewGroup.LayoutParams.WRAP_CONTENT );
relativeParams.leftMargin = (x + Utils.dpToPx(35, density)) + (col * Utils.dpToPx(99, density)); relativeParams.topMargin = (y + Utils.dpToPx(162, density)) + (row * Utils.dpToPx(99, density));
rootLayout.addView(dotImgView,relativeParams);
|
参考文章
六大布局之RelativeLayout