一. 前言
BroadcastReceiver
是 Android
四大组件之一,是一种广泛运用在应用程序之间异步传输信息的机制。
Broadcast
本质上是一个Intent
对象,差别在于Broadcast
可以被多个 BroadcastReceiver
处理。BroadcastReceiver
是一个全局监听器,通过它的 onReceive()
可以过滤用户想要的广播收音机,进而进行其它操作。
BroadcastReceiver
默认是在主线程中执行,如果onReceiver()
方法处理事件超过10s
,则应用将会发生ANR(Application Not Responding)
,此时,如果建立工作线程并不能解决此问题,因此建议:如处理耗时操作,请用 Service
代替。
BroadcastReceiver
的主要声明周期方法onReceiver()
,此方法执行完之后,BroadcastReceiver
实例将被销毁。
二. BroadcastReceiver的生命周期
1. Register
2. SendBroadcast
3. onReceive
4. unRegister
三. BroadcastReceiver的注册
1. 静态注册
在AndroidManifest.xml文件中类似于其他组件的注册方式。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28
| <?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="swu.xl.broadcastreceiver">
<application android:allowBackup="true" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:roundIcon="@mipmap/ic_launcher_round" android:supportsRtl="true" android:theme="@style/AppTheme"> <activity android:name=".MainActivity"> <intent-filter> <action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> <receiver android:name=".ShowBroadcastReceiver"> <intent-filter> <action android:name="SHOW_ACTION"/> </intent-filter> </receiver> </application>
</manifest>
|
2. 动态注册
1 2 3 4 5 6 7
| IntentFilter intentFilter = new IntentFilter(); intentFilter.addAction(SHOW_ACTION); registerReceiver(showBroadcastReceiver,intentFilter);
unregisterReceiver(showBroadcastReceiver);
|
3. 两者的区别
静态注册是在AndroidManifest.xml文件中,所以一般情况下是不会移除的,比较占用资源。
动态注册于onStart移除于onStop,所以建议使用动态注册。
四. 简单的动态注册实例
1. 继承于BroadcastReceiver的类
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| public class ShowBroadcastReceiver extends BroadcastReceiver { @Override public void onReceive(Context context, Intent intent) { if (intent != null){ if (TextUtils.equals(intent.getAction(),MainActivity.SHOW_ACTION)){ String toast_message = intent.getStringExtra("toast"); Toast.makeText(context, toast_message, Toast.LENGTH_SHORT).show(); } } } }
|
2. 注册,发送
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43
| public class MainActivity extends AppCompatActivity {
public static final String SHOW_ACTION = "SHOW_ACTION";
public ShowBroadcastReceiver showBroadcastReceiver = new ShowBroadcastReceiver();
@Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main);
findViewById(R.id.send).setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { Intent intent = new Intent(); intent.setAction(SHOW_ACTION); intent.putExtra("toast","消息来了"); sendBroadcast(intent); } }); }
@Override protected void onStart() { super.onStart();
IntentFilter intentFilter = new IntentFilter(); intentFilter.addAction(SHOW_ACTION); registerReceiver(showBroadcastReceiver,intentFilter); }
@Override protected void onStop() { super.onStop();
unregisterReceiver(showBroadcastReceiver); } }
|
3. 运行效果
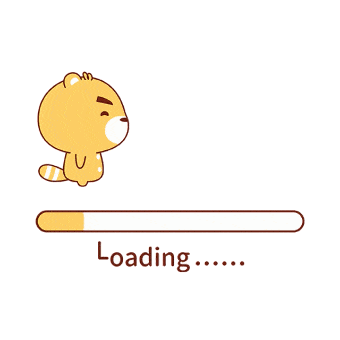
4. 源码
BroadcastRecevier
五. 广播的发送
1. 发送无序广播
发送无序广播在Android
中很常见,是一种一对多的关系,主要通过 sendBroadcast(intent);
发送广播。
2. 发送有序广播
广播在Android
是有优先级的,优先级高的广播可以终止或修改广播内容。发送有序广播的方法如下sendOrderedBroadcast(intent,"str_receiver_permission");
六. 接受系统广播的方法(以开机广播为例)
1. 实现BootReceiverMethod 继承 BroadcastReceiver
1 2 3 4 5 6 7 8
| public class BootReceiverMethod extends BroadcastReceiver { @Override public void onReceive(Context context, Intent intent) { Intent mStartIntent = new Intent(context, StartServiceMethods.class); context.startService(mStartIntent); } }
|
2. 在Androidmainfest.xml 声明组件信息,并过滤开机完成 Action
1 2 3 4 5 6 7 8
| <receiver android:name=".component.BroadcastReceiver.BootReceiverMethod" android:enabled="true" android:exported="true"> <intent-filter> <action android:name="android.intent.action.BOOT_COMPLETED" /> </intent-filter> </receiver>
|
3. 声明权限
1
| <uses-permission android:name="android.permission.RECEIVE_BOOT_COMPLETED" />
|
参考文章
Broadcast 使用详解