一. 先看下面的布局
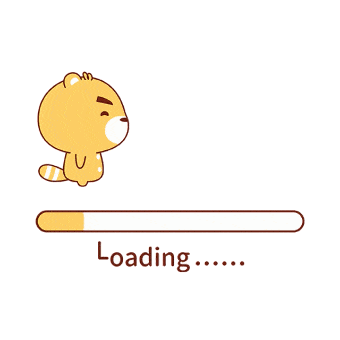
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37
| <?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".FirstActivity" android:orientation="horizontal">
<TextView android:layout_width="0dp" android:layout_height="48dp" android:layout_weight="1" android:gravity="center" android:text="111111111111" android:background="#44ff0000" />
<TextView android:layout_width="0dp" android:layout_height="48dp" android:layout_weight="2" android:gravity="center" android:text="2" android:background="#4400ff00" />
<TextView android:layout_width="0dp" android:layout_height="48dp" android:layout_weight="3" android:gravity="center" android:text="3" android:background="#440000ff" />
</LinearLayout>
|
在水平方向的线性布局中,有三个TextView,layout_width
都是0dp,layout_weight
分别为1,2,3,所以这三个TextView应该按1:2:3的比例分布。
但是我们从示意图看到第一个TextView却没有和另外两个对齐,这是为什么呢?仔细看就能发现,虽然三个TextView不是对齐的,但是第一行的文本是对齐的。
我们只需将 LinearLayout 的 android:baselineAligned
属性设置为 false 即可,android:baselineAligned
表示是否以文本基准线对齐。
二. 修改不以文本基准线对齐后
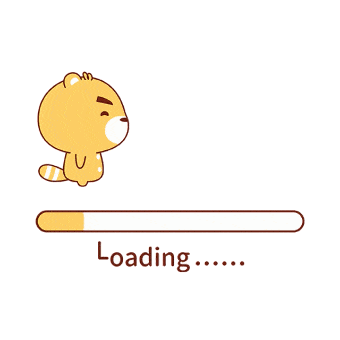
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39
| <?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".FirstActivity" android:orientation="horizontal"
android:baselineAligned="false">
<TextView android:layout_width="0dp" android:layout_height="48dp" android:layout_weight="1" android:gravity="center" android:text="111111111111" android:background="#44ff0000" />
<TextView android:layout_width="0dp" android:layout_height="48dp" android:layout_weight="2" android:gravity="center" android:text="2" android:background="#4400ff00" />
<TextView android:layout_width="0dp" android:layout_height="48dp" android:layout_weight="3" android:gravity="center" android:text="3" android:background="#440000ff" />
</LinearLayout>
|
三. 做一些修改后
假如把第一个 TextView 的 layout_width
改为 wrap_content
,会出现什么情况呢?
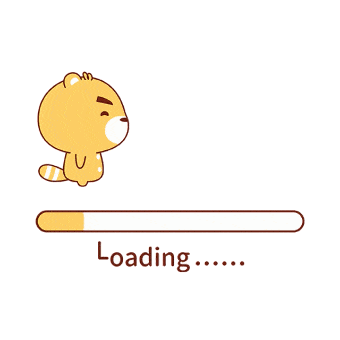
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39
| <?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".FirstActivity" android:orientation="horizontal" android:baselineAligned="false">
<TextView android:layout_width="wrap_content" android:layout_height="48dp" android:layout_weight="1" android:gravity="center" android:text="111111111111" android:background="#44ff0000" />
<TextView android:layout_width="0dp" android:layout_height="48dp" android:layout_weight="2" android:gravity="center" android:text="2" android:background="#4400ff00" />
<TextView android:layout_width="0dp" android:layout_height="48dp" android:layout_weight="3" android:gravity="center" android:text="3" android:background="#440000ff" />
</LinearLayout>
|
发现三个 TextView 并没有再按照 1:2:3
的比例进行分配.其实,layout_weight
属性是先按控件声明的尺寸进行分配,然后将剩余的尺寸按layout_weight
的比例进行分配。
设屏幕总宽度为 sum,第一个TextView声明的尺寸为 x,那么:
第一个 TextView 的宽度为: x + (sum - x) * 1/6
第二个 TextView 的宽度为: (sum - x) * 2/6
第三个 TextView 的宽度为: (sum - x) * 3/6
四. 验证结论的修改
为了证实这个结论,接下来继续修改布局,将三个 TextView 的 layout_width
都设置为 match_parent
,把 layout_weight
分别设置为1,2,2
。
设屏幕总宽度为sum,由于这三个 TextView 声明的尺寸都是 match_parent
,也就是sum,则控件声明的尺寸为 sum * 3。那么:
第一个 TextView 的宽度为: sum + (sum - sum * 3) * 1/5
第二个 TextView 的宽度为: sum + (sum - sum * 3) * 2/5
第三个 TextView 的宽度为: sum + (sum - sum * 3) * 2/5
最终三个 TextView 的宽度比例 是:3 : 1 : 1。下面的结果也证实了我们的猜测。
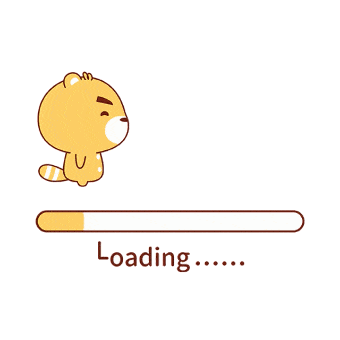
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39
| <?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".FirstActivity" android:orientation="horizontal" android:baselineAligned="false">
<TextView android:layout_width="match_parent" android:layout_height="48dp" android:layout_weight="1" android:gravity="center" android:text="111111111111" android:background="#44ff0000" />
<TextView android:layout_width="match_parent" android:layout_height="48dp" android:layout_weight="2" android:gravity="center" android:text="2" android:background="#4400ff00" />
<TextView android:layout_width="match_parent" android:layout_height="48dp" android:layout_weight="2" android:gravity="center" android:text="3" android:background="#440000ff" />
</LinearLayout>
|
参考文章
Android常用布局属性解析 – Layout_weight