一. 前言
模仿系统的XLToolBar。
Github地址:XLToolBar
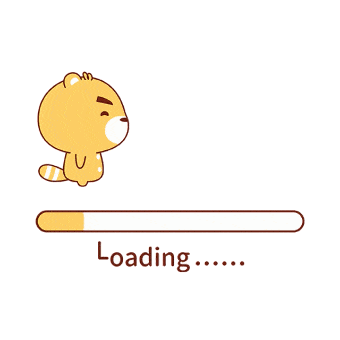
二. 自定义View
1. 自定义用到的属性
1 2 3 4 5 6 7 8 9
| private Button left_btn;
private Button logo_btn;
private Button right_btn;
private int layout_id;
|
2. 在values文件夹下自定义属性
1 2 3 4 5 6 7
| <?xml version="1.0" encoding="utf-8"?> <resources> <declare-styleable name="XLToolBar"> <attr name="layout_id" format="reference"/> </declare-styleable> </resources>
|
3. 创建构造方法
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49
|
public XLToolBar(Context context, int layout_id) { super(context);
this.layout_id = layout_id;
init(); }
public XLToolBar(Context context, @Nullable AttributeSet attrs) { super(context, attrs);
if (attrs != null){ TypedArray typedArray = context.obtainStyledAttributes(attrs, R.styleable.XLToolBar);
layout_id = typedArray.getResourceId(R.styleable.XLToolBar_layout_id,layout_id);
typedArray.recycle(); }
init(); }
private void init() { View inflate = LayoutInflater.from(getContext()).inflate(R.layout.tool_bar_layout, this);
left_btn = inflate.findViewById(R.id.left); logo_btn = inflate.findViewById(R.id.logo); right_btn = inflate.findViewById(R.id.right); }
|
4. 默认的布局文件
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42
| <?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:background="#F9F5F8">
<Button android:id="@+id/left" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="左边" android:textSize="17sp" android:textColor="#4A3740" android:background="@null" android:layout_centerVertical="true" android:layout_marginStart="-8dp" />
<Button android:id="@+id/logo" android:layout_width="wrap_content" android:layout_height="wrap_content" android:background="@drawable/logo" android:layout_centerInParent="true" />
<Button android:id="@+id/right" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="右边" android:textSize="17sp" android:textColor="#4A3740" android:background="@null" android:layout_centerVertical="true" android:layout_alignParentEnd="true" android:layout_marginEnd="-8dp" />
</RelativeLayout>
|
想要传入自定义布局时,布局中一定要有三个Button,且其id依次是left,logo,right。
三. 具体的使用
首先需要添加依赖,添加依赖之后,在布局文件中这样调用。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| <?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity" android:background="@color/colorAccent">
<swu.xl.xltoolbar.XLToolBar android:id="@+id/tool_bar" android:layout_width="match_parent" android:layout_height="45dp" />
</RelativeLayout>
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| public class MainActivity extends AppCompatActivity {
@Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main);
XLToolBar toolBar = findViewById(R.id.tool_bar); toolBar.getLeft_btn().setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { Toast.makeText(MainActivity.this, "左边按钮被点击了", Toast.LENGTH_SHORT).show(); } }); toolBar.getRight_btn().setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { Toast.makeText(MainActivity.this, "右边按钮被点击了", Toast.LENGTH_SHORT).show(); } }); } }
|
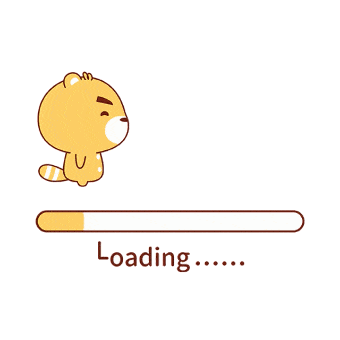