一. 继承关系
1 2 3 4 5 6
| java.lang.Object ↳android.view.View ↳android.view.ViewGroup ↳android.widget.FrameLayout ↳android.widget.HorizontalScrollView ↳com.google.android.material.tabs.TabLayout
|
Tablayout继承自HorizontalScrollView,用作页面切换指示器,因使用简便功能强大而广泛使用在App中。
需要注意一点,AndroidStuid默认是没有添加对 TableLayout的依赖的。
添加的方式有两种:
- 点击下载的按钮,TabLayout,系统就会自动帮你添加依赖。
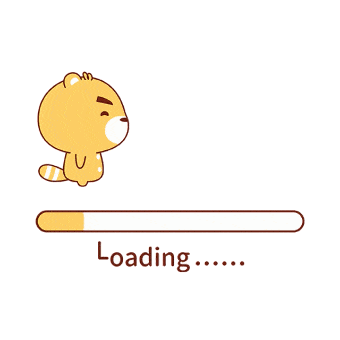
- 主动添加依赖,不推荐。
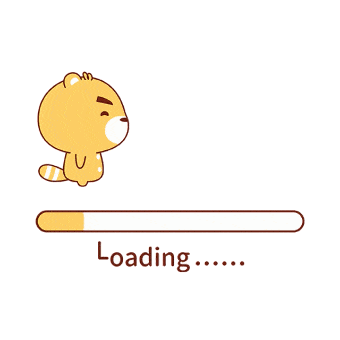
二. TabLayout的Xml属性
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47
| app:tabIndicatorColor: app:tabIndicatorHeight: app:tabIndicatorFullWidth:
android:background:
app:tabBackground:
app:tabGravity:
app:tabContentStart:
app:tabTextColor: app:tabSelectedTextColor:
app:tabTextAppearance:tab的字体样式style
app:tabMode:
app:tabRippleColor:
app:tabPadding: app:tabPaddingTop: app:tabPaddingBottom: app:tabPaddingStart: app:tabPaddingEnd:
|
三. TabLayout的方法
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67
| addOnTabSelectedListener(TabLayout.OnTabSelectedListener listener)
clearOnTabSelectedListeners()
addTab(TabLayout.Tab tab,boolean setSelected) addTab(TabLayout.Tab tab,int position) addTab(TabLayout.Tab tab) addTab(TabLayout.Tab tab,int position,boolean setSelected)
addView(View child,int index) addView(View child) addView(View child,ViewGroup.LayoutParams params) addView(View child,int index,ViewGroup.LayoutParams params)
int getSelectedTabPosition()
TabLayout.Tab getTabAt(int index)
int getTabCount()
setTabGravity(int gravity)
int getTabGravity()
setTabMode(int mode)
int getTabMode()
ColorStateList getTabTextColors()
removeAllTabs()
从布局中删除选项卡。 removeTab(TabLayout.Tab tab) removeTabAt(int position)
setScrollPosition(int position,float positionOffset,boolean updateSelectedText)
setSelectedTabIndicatorHeight(int height)
setSelectedTabIndicatorColor(int color)
setTabTextColors(int normalColor,int selectedColor) setTabTextColors(ColorStateList textColor)
setupWithViewPager(ViewPager viewPager)
setupWithViewPager(ViewPager viewPager,boolean autoRefresh)
|
四. 各种样式的例子
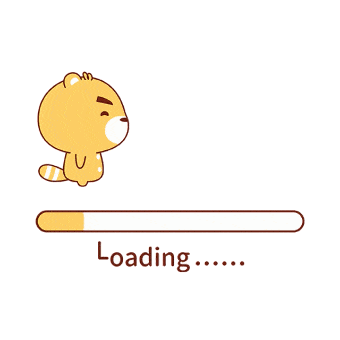
Tablayout-Github
1. 正常使用
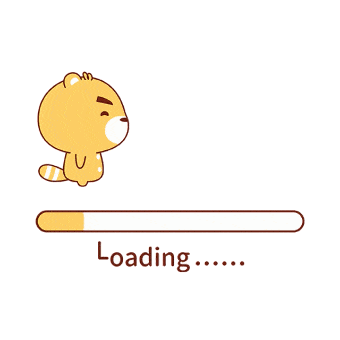
① 设置字体的样式
1 2 3 4 5 6 7 8 9
|
<style name="MyTextAppearance" parent="TextAppearance.AppCompat"> <!--true: 英文默认全大写 false: 英文分大小写 --> <item name="android:textAllCaps">true</item> <item name="android:textSize">20sp</item> <item name="android:textStyle">bold</item> </style>
|
② 设置背景的样式
1 2 3 4 5 6 7 8 9 10 11 12
|
<?xml version="1.0" encoding="utf-8"?> <selector xmlns:android="http://schemas.android.com/apk/res/android"> <item android:drawable="@color/colorRed" android:state_selected="false"/> <item android:drawable="@color/colorGreen" android:state_selected="true"/> </selector>
|
③ 使用
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| <com.google.android.material.tabs.TabLayout android:id="@+id/tab_1" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginTop="20dp"
app:tabBackground="@drawable/my_tab_background"
app:tabPadding="0dp"
app:tabTextColor="#ffffff" app:tabSelectedTextColor="#000000"
app:tabIndicatorHeight="10sp" app:tabIndicatorColor="#000000"
app:tabTextAppearance="@style/MyTextAppearance" />
|
1 2 3 4 5 6 7
|
TabLayout tabLayout1 = findViewById(R.id.tab_1); tabLayout1.addTab(tabLayout1.newTab().setText("Tab1")); tabLayout1.addTab(tabLayout1.newTab().setText("Tab2")); tabLayout1.addTab(tabLayout1.newTab().setText("Tab3"));
|
2. 不设置指示线
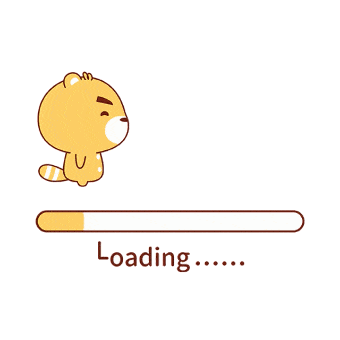
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| <com.google.android.material.tabs.TabLayout android:id="@+id/tab_2" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginTop="20dp"
app:tabBackground="@drawable/my_tab_background"
app:tabPadding="0dp"
app:tabTextColor="#ffffff" app:tabSelectedTextColor="#000000"
app:tabIndicatorHeight="0sp" app:tabIndicatorColor="#000000" app:tabIndicatorFullWidth="true"
app:tabTextAppearance="@style/MyTextAppearance" />
|
1 2 3 4 5 6 7
|
TabLayout tabLayout2 = findViewById(R.id.tab_2); tabLayout2.addTab(tabLayout2.newTab().setText("Tab1")); tabLayout2.addTab(tabLayout2.newTab().setText("Tab2")); tabLayout2.addTab(tabLayout2.newTab().setText("Tab3"));
|
3. 指示线宽度
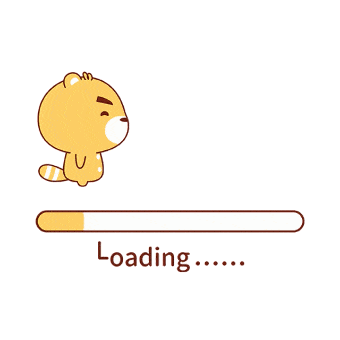
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| <com.google.android.material.tabs.TabLayout android:id="@+id/tab_3" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginTop="20dp"
app:tabBackground="@drawable/my_tab_background"
app:tabPadding="0dp"
app:tabTextColor="#ffffff" app:tabSelectedTextColor="#000000" app:tabIndicatorHeight="10sp" app:tabIndicatorFullWidth="false"
app:tabIndicatorColor="#000000"
app:tabTextAppearance="@style/MyTextAppearance" />
|
1 2 3 4 5 6 7
|
final TabLayout tabLayout3 = findViewById(R.id.tab_3); tabLayout3.addTab(tabLayout3.newTab().setText("Tab1")); tabLayout3.addTab(tabLayout3.newTab().setText("Tab2")); tabLayout3.addTab(tabLayout3.newTab().setText("Tab3"));
|
4. 添加图标
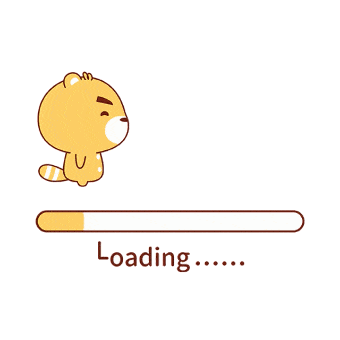
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| <com.google.android.material.tabs.TabLayout android:id="@+id/tab_4" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginTop="20dp"
app:tabBackground="@drawable/my_tab_background"
app:tabPadding="0dp"
app:tabTextColor="#ffffff" app:tabSelectedTextColor="#000000" app:tabIndicatorHeight="10sp" app:tabIndicatorFullWidth="true"
app:tabIndicatorColor="#000000"
app:tabTextAppearance="@style/MyTextAppearance" />
|
1 2 3 4 5 6 7 8 9 10
|
final TabLayout tabLayout4 = findViewById(R.id.tab_4); tabLayout4.addTab(tabLayout4.newTab().setText("Tab1")); tabLayout4.addTab(tabLayout4.newTab().setText("Tab2")); tabLayout4.addTab(tabLayout4.newTab().setText("Tab3")); for (int i = 0; i < tabLayout4.getTabCount(); i++) { tabLayout4.getTabAt(i).setIcon(R.mipmap.ic_launcher_round); }
|
5. 自定义图标位置
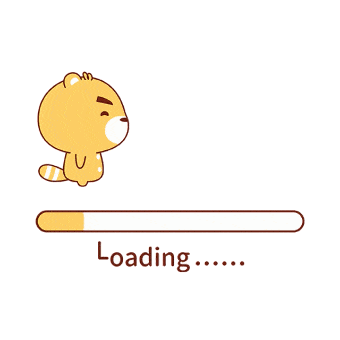
① 自定义的布局xml文件
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26
|
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="wrap_content" android:layout_height="48dp" android:orientation="horizontal" android:gravity="center"> <ImageView android:id="@+id/imgView" android:layout_width="24dp" android:layout_height="24dp" />
<TextView android:id="@+id/textView" android:layout_width="wrap_content" android:layout_height="match_parent" android:gravity="center" android:textSize="20sp" android:textStyle="bold" android:layout_marginStart="8dp" />
</LinearLayout>
|
② 加载自定义布局,绑定数据
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
|
private View myTabView(int position,String[] texts,int[] resources){ View tabView = LayoutInflater.from(this).inflate(R.layout.tab_layout, null);
ImageView imageView = tabView.findViewById(R.id.imgView); TextView textView = tabView.findViewById(R.id.textView);
imageView.setImageResource(resources[position]); textView.setText(texts[position]);
return tabView; }
|
③ 使用
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
| <com.google.android.material.tabs.TabLayout android:id="@+id/tab_5" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginTop="20dp"
app:tabBackground="@drawable/my_tab_background"
app:tabPadding="0dp"
app:tabTextColor="#ffffff" app:tabSelectedTextColor="#000000" app:tabIndicatorHeight="10sp" app:tabIndicatorFullWidth="true"
app:tabIndicatorColor="#000000"
app:tabTextAppearance="@style/MyTextAppearance" />
|
1 2 3 4 5 6 7 8 9 10 11 12
|
final TabLayout tabLayout5 = findViewById(R.id.tab_5); String[] texts = {"Tab1","Tab2","Tab3"}; int[] resources = {R.mipmap.ic_launcher_round,R.mipmap.ic_launcher_round,R.mipmap.ic_launcher_round}; for (int i = 0; i < texts.length; i++) { tabLayout5.addTab(tabLayout5.newTab()); } for (int i = 0; i < tabLayout5.getTabCount(); i++) { tabLayout5.getTabAt(i).setCustomView(myTabView(i,texts,resources)); }
|
6. 滚动模式
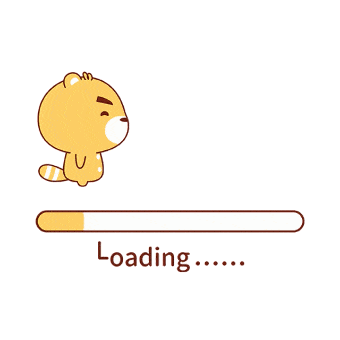
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| <com.google.android.material.tabs.TabLayout android:id="@+id/tab_6" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginTop="20dp"
android:background="#fbf4f2"
app:tabPadding="0dp"
app:tabTextColor="#000000" app:tabSelectedTextColor="#ff7a61" app:tabIndicatorHeight="2sp" app:tabIndicatorFullWidth="true"
app:tabIndicatorColor="#ff7a61"
app:tabMode="scrollable" />
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
|
final TabLayout tabLayout6 = findViewById(R.id.tab_6); tabLayout6.addTab(tabLayout6.newTab().setText("Tab1")); tabLayout6.addTab(tabLayout6.newTab().setText("Tab2")); tabLayout6.addTab(tabLayout6.newTab().setText("Tab3")); tabLayout6.addTab(tabLayout6.newTab().setText("Tab4")); tabLayout6.addTab(tabLayout6.newTab().setText("Tab5")); tabLayout6.addTab(tabLayout6.newTab().setText("Tab6")); tabLayout6.addTab(tabLayout6.newTab().setText("Tab7")); tabLayout6.addTab(tabLayout6.newTab().setText("Tab8")); tabLayout6.addTab(tabLayout6.newTab().setText("Tab9")); tabLayout6.addTab(tabLayout6.newTab().setText("Tab10")); tabLayout6.addTab(tabLayout6.newTab().setText("Tab11")); tabLayout6.addTab(tabLayout6.newTab().setText("Tab12"));
|
五. 结合 ViewPagger
参考:ViewPager
六. 完善的内容
1. 静态添加 TabItem 的当时
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| <android.support.design.widget.TabLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content">
<android.support.design.widget.TabItem
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Tab1"/>
...
</android.support.design.widget.TabLayout>
|
参考文章
第三方库:XTabLayout
手把手教你如何在Android Studio 中配置Android Design Support Library
Tablayout使用全解,一篇就够了
TabLayout的属性介绍
Design库-TabLayout属性详解
设置tablayout的字体大小
android TabLayout 选中改变背景
TabLayout设置下划线(Indicator)宽度
Android开发TabLayout取消水波纹效果以及按下阴影效果
android appcompat_v7 去掉点击效果或者自定义水波纹颜色
Android TabLayout 与ViewPager滑动页面的实现